Coroutines: Builders
Quick save... Quick load
Even though coroutines attempt to mask our direct use of threads, we ultimately still rely on the threads to run our suspended functions. This is so we can use the OS's scheduling and execution features.
Coroutines act as a container to run suspended functions in a thread. Remember, the power of suspended functions come from their ability to be quickly saved, loaded and executed. Read more
The way Kotlin is set up, we can only run suspended functions inside a coroutine.
Coroutines are created by using one of these three builders: (I will explain scope later on)
Launch
scope.launch{
suspendedFunction1()
}
This is used when we don't need the coroutine to provide us with a result. When we run this with other code, a new line of concurrent code is created. Here is an adaptation of the example from the book:
fun main() {
GlobalScope.launch {
delay(300)
println("Coroutine activated")
}
println("Main branch activated")
Thread.sleep(5000)
}
The print statement for "Main branch activated" is run immediately after the creation of the coroutine. There isn't any delay. The lambda function for the coroutine is run as soon as it's created. In this instance, we've created a short 300ms delay. The output here would be:
Main branch activated
Coroutine activated
I've created a graphical representation of these events below. This may be easier to see how we now have two parallel lines of code running on their own timelines.
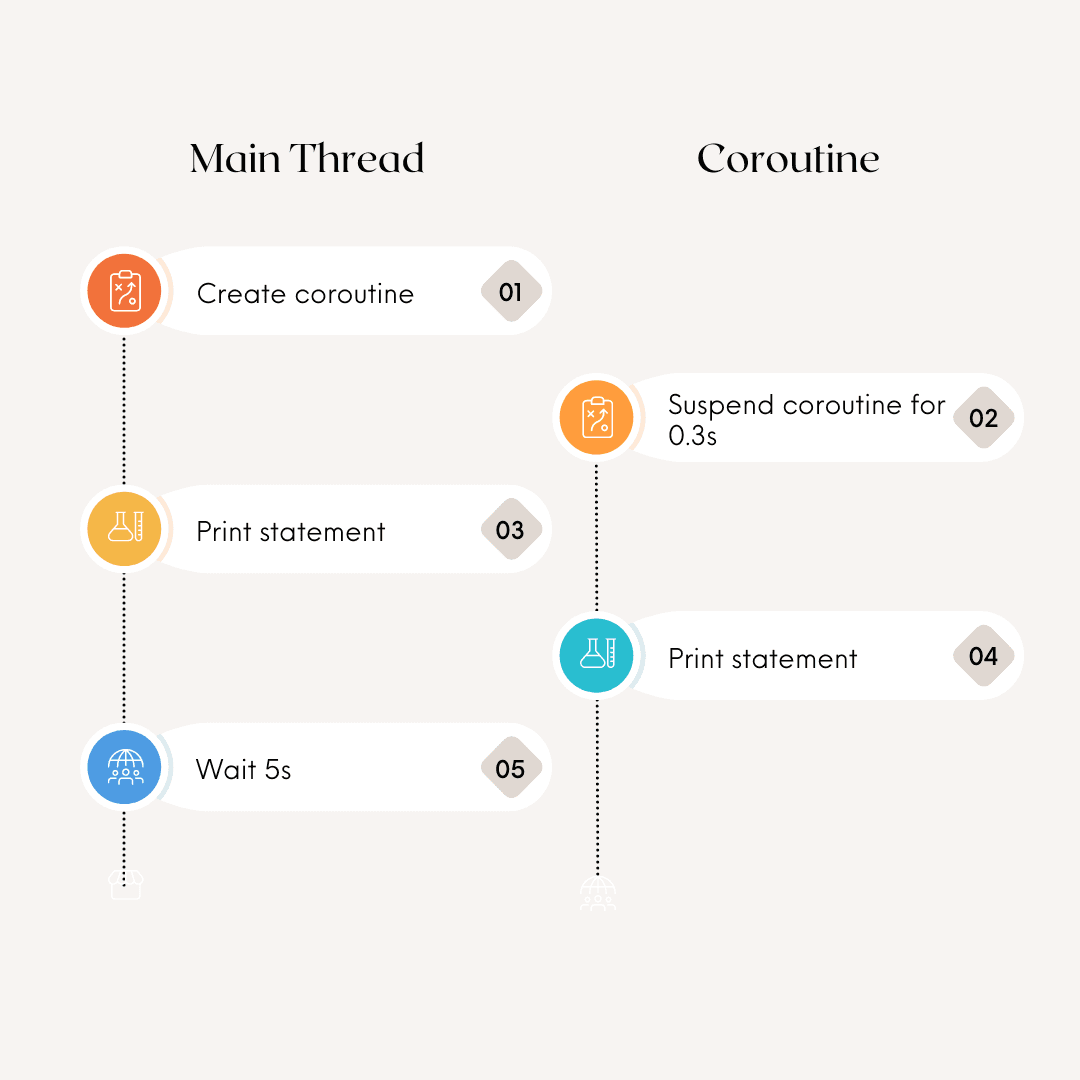
Async
scope.async {
suspendedFunction1()
}
I will go into this more in detail at a later post.
Run Blocking
runBlocking {
suspendedFunction1()
}
The launch {}
builder doesn't block the thread when creating the coroutine so we have to solve it by putting a delay or a join (explained later in Jobs).
The runBlocking
builder blocks the thread as the coroutine is created. The thread then continues when the coroutine has completed.